What is Gatsby?
Gatsby is an open-source framework that combines functionality from React, GraphQL and Webpack into a single tool for building static websites and apps. Owing to the fast performance of the sites it powers, impressive out-of-the-box features like code splitting, and friendly developer experience, Gatsby is fast becoming a staple of modern web development.
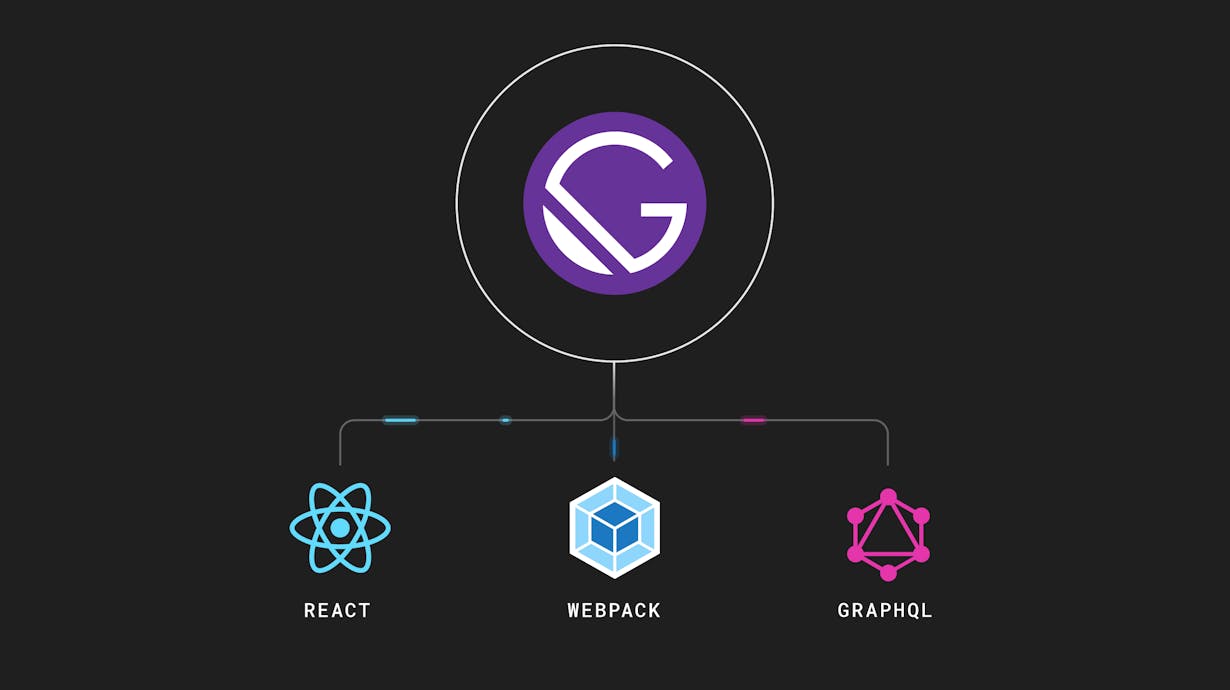
You’re currently visiting a website powered by Gatsby, and there is a reason for that. Not long ago, we at mParticle decided to invest in improving the experience of our website. We wanted pages to load faster while adhering to the highest standards of accessibility. We wanted to put extensible tools in the hands of our developers to build and expand upon the site well into the future. We considered what we had, where we wanted to go, and the options we had for getting there.
In the end, we decided to overhaul our site with Gatsby, and we’re glad we did. To learn more specifics about our particular use case with Gatsby, here's a post detailing our website overhaul. Here, we'll explore the framework itself––what it is, what it’s built on, and what differentiates it from other tools in the world of static web applications.
Bringing clarity to the buzzword buffet
Gatsby is a creature of the modern web––the world of “JAMstack” architecture, static site generators and other newfangled concepts that make websites load faster and run more smoothly. In order to understand Gatsby, we need to pan out slightly and define some of the technologies it rests on, many of which have been shaping the web and web development for the last several years.
Static site generators
Since Gatsby is a static site generator at its core, let’s explore this concept before diving into anything else. If you come across a plain HTML page that has been sitting on a server since the mid 1990s, you’ve found a static website. So what, you ask, is particularly innovative about something that can generate static content on the web?
The special ingredient that static site generators bring to the table is dynamic content loading. In a traditional database-driven content management system like Wordpress or Drupal, a software layer receives resource requests from a client, merges the appropriate templates and content files, then serves a complete HTML page in response. Static site generators, however, separate the content from the templates that will display them. They generate HTML pages at build time by combining templates with content fetched from an external API, then deploy these pages to a web server which responds with rendered HTML.
By building and serving web pages in this manner, static site generators offer several advantages. Most importantly, by eliminating the latency associated with databases, the speed and reliability of websites built on this architecture is greatly improved. Not only do static sites load faster in the browser, but the risk of traffic spikes toppling the database is eliminated, as is the need to manage database server software and backups.
Another key advantage of static site generators is that you are not tied to a single CMS or database to serve your site’s content. Instead, you can pull in content from any external API that is compatible with the static site generator you are using. Though some static site generators are limited to only loading content from Markdown files, others (including Gatby) are more flexible and allow for a wider variety of sources to comprise the data layer––more on this later.
Finally, owing to these significant performance gains, websites running on static site generators tend to realize overall usability enhancements as well as significant advantages in terms of SEO. This is now more important than ever, as Google has announced that its upcoming Page Experience Update will use three key load metrics to assess the user experience of a given webpage, and that this will have a direct impact on page rankings.
The “JAMstack”
Static site generators enable a type of web architecture referred to as the “JAMstack,” a portmanteau of “JavaScript, APIs, and Markup” and a modern take on structuring the different resources required to host and serve web content.
Let’s look at what each component of “JAM” brings to the table:
- JavaScript handles the dynamic functionalities involved in combining the data and content with UI templates. JAMstack architecture places no restrictions on the frameworks or libraries that can be used to accomplish this.
- APIs are the piece of the puzzle that allow traditional server-side operations to be abstracted away, either by third-party services (like a content delivery network, a CMS with external plugins), or a custom-built function.
- Markup: The “M” in JAM refers to the fact that these websites use Markdown files as the source from which to serve static HTML files. In the case of Gatsby, these Markdown files are fetched via plugins connected to other APIs, CMSs or databases.
Gatsby’s take on the JAMstack
If we are to think about Gatsby as a tool for building JAMstack sites, React is the “J” in Gatsby’s “JAM.” React components are highly modular and reusable, so they are ideal for serving as wrappers for dynamic content. Gatsby is designed to operate almost entirely like a normal React application, so if you’re familiar with using React, you’ll feel right at home developing in a Gatsby application.
React is the “J” in “JAM”
The function of a component within a Gatsby application depends on its location within the filesystem. Components under src/components are simple page components, which generate pages at URLs based on their filenames––e.g. src/pages/about.js would generate mywebsite.com/about. Page template components are similar to page components, though they are housed under src/templates, and are capable of querying GraphQL to retrieve markdown data that is then populated in the page they generate. Page template components are useful for generating resources within a site that will maintain a consistent interface layout but display different content, such as blog posts, user profile pages, and dashboards.
GraphQL ties APIs to components
Like React, GraphQL was invented at Facebook, so it is understandably one of the most powerful and flexible methods for loading data into React components. As a query language (hence the “QL”), GraphQL works in a manner similar to SQL in that the user describes the necessary data in a “query,” and it is returned in a response.
In some ways, however, GraphQL syntax is simpler and more declarative than SQL’s. For example, consider the following query:
const query = graphql
query BlogPostQuery {
site {
title
content
}
}
The data that this query returns would maintain the same structure as the query itself:
data: {
site: {
title: "Seven tips for sprucing up your living room"
content : "Here is the content for this blog post about sprucing up your living room.”
}
}
Flexibility beyond Markup alone
Unlike some static site generators which are only able to ingest Markup files as to build the content layer of components, Gatsby sites come with the flexibility to ingest a variety of data types from numerous sources. The most popular choice for content management are “headless” CMSes, which are content management software tools that are not tied to a specific set of web technologies for rendering data.
Contentful, DatoCMS, Prismic, Sanity and Strapi are some of the top headless CMSes that Gatsby developers reach for. Gatsby’s plugin documentation lists additional CMS options, as well as popular tools for CSS preprocessing, UI component libraries, ecommerce functionality, and analytics.
Getting started with Gatsby
Gatsby makes it very easy to get up and running with a “hello world” boilerplate project by running simple CLI commands. Assuming you have Node installed locally, install Gatsby globally:
npm install -g gatsby-cli
Now you can create a local directory and remote repository where you’d like your project to live, and run the following:
gatsby new [SITE_DIRECTORY_NAME] [URL_OF_STARTER_GITHUB_REPO]
This will bootstrap all of the files and project architecture you need for a Gatsby project. Once it has generated, open up the project in your IDE of choice, navigate to src/pages/index.js, and you’ll see the world’s simplest React component serving as your site’s homepage:
export default () => <div>Hello World!</div>
Now you’re ready to take advantage of everything the Gatsby ecosystem has to offer and build and deploy a highly performant static site. You can add new pages with Markdown, add components to your Markdown with MDX, experiment with sourcing and querying data in your site, and much more.
Send customer and event data from your Gatsby site to your Customer Data Platform
Once you get a website up and running with Gatsby, the mParticle Gatsby plugin makes it easy to connect your site to mParticle and start collecting user and event data. In your project’s root directory, run a quick NPM install:
npm i @mparticle/gatsby-plugin-mparticle
Then, in your gatsby-js.config, add:
module.exports = {
plugins: [
// Other plugins here...
{
resolve: '@mparticle/gatsby-plugin-mparticle',
options: {
apiKey: 'YOUR APP KEY',
logPageViews: true or false,
config: {
'Your mParticle config object here, if needed e.g. isDevelopmentMode',
},
}
}
]
}
Hopefully now you have a good understanding of what GatsbyJS is, and the place it occupies in the ecosystem of modern web development tools. Soon, we’ll explore how and why we chose to migrate this very website to a Gatsby build, and the wide variety of gains we’ve seen after having done so.